How To Add A Single Item To An Array In Java
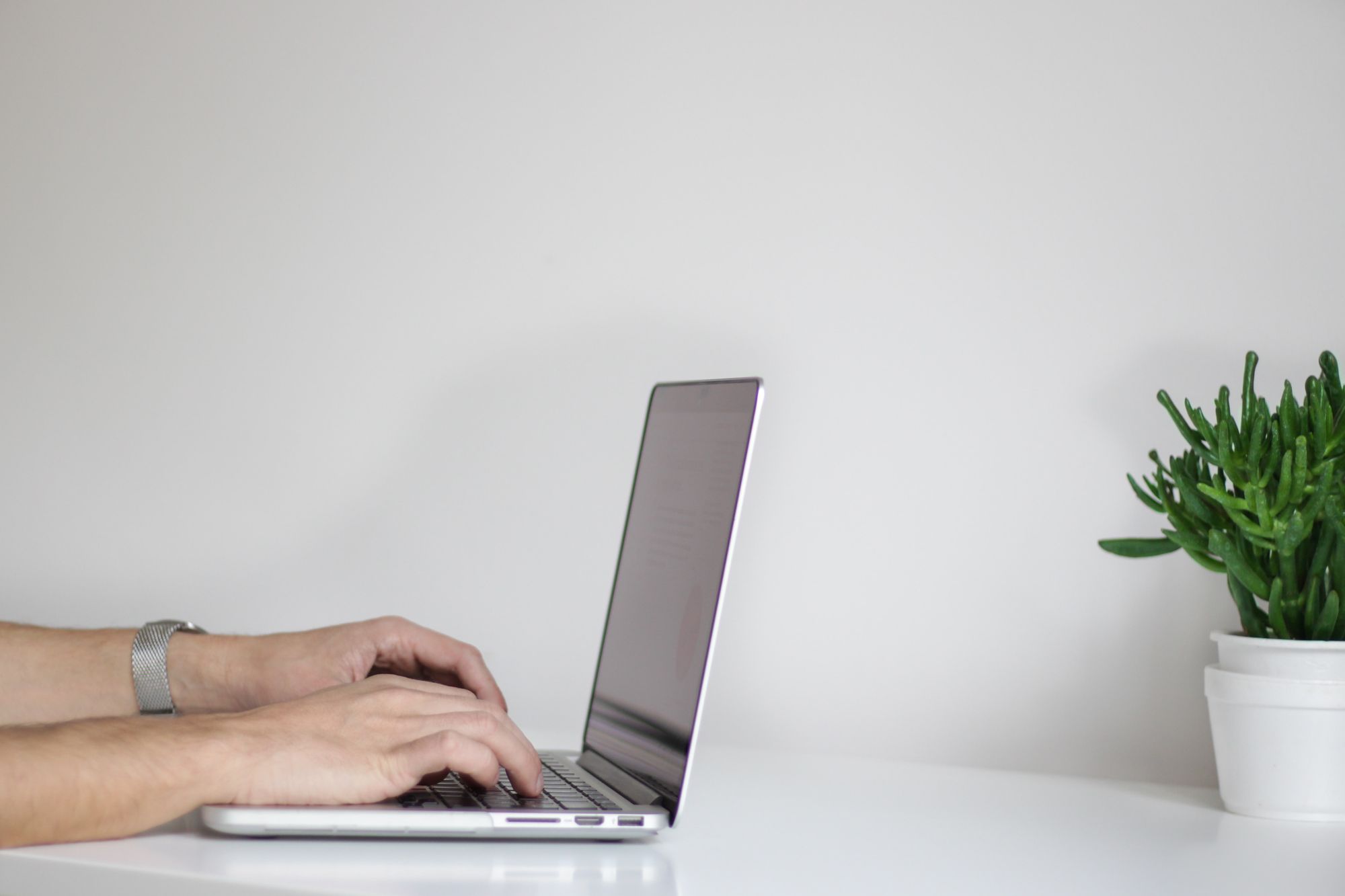
In this article, y'all'll larn about the .append()
method in Python. You'll as well run into how .append()
differs from other methods used to add elements to lists.
Allow's get started!
What are lists in Python? A definition for beginners
An array in programming is an ordered drove of items, and all items need to be of the same information type.
All the same, unlike other programming languages, arrays aren't a born data structure in Python. Instead of traditional arrays, Python uses lists.
Lists are essentially dynamic arrays and are one of the most mutual and powerful data structures in Python.
You tin can think of them as ordered containers. They shop and organize like kind of related data together.
The elements stored in a list can be of whatsoever data blazon.
There can exist lists of integers (whole numbers), lists of floats (floating signal numbers), lists of strings (text), and lists of any other congenital-in Python data blazon.
Although it is possible for lists to concord items of just the same information type, they are more than flexible than traditional arrays. This ways that in that location tin exist a diversity of unlike data types inside the same list.
Lists have 0 or more items, meaning at that place can also be empty lists. Inside a listing at that place tin also exist indistinguishable values.
Values are separated by a comma and enclosed in square brackets, []
.
How to create lists in Python
To create a new listing, outset requite the list a name. Then add together the assignment operator(=
) and a pair of opening and closing square brackets. Inside the brackets add the values you want the list to contain.
#create a new list of names names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #print the list to the console print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny']
How lists are indexed in Python
Lists maintain an order for each item.
Each item in the collection has an its own alphabetize number, which y'all can utilise to access the particular itself.
Indexes in Python (and every other modern programming language) kickoff at 0 and increase for every particular in the list.
For instance, the listing created earlier on had iv values:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"]
The kickoff value in the list, "Jimmy", has an index of 0.
The second value in the list, "Timmy", has an index of one.
The 3rd value in the listing, "Kenny", has an index of 2.
The fourth value in the list, "Lenny", has an index of 3.
To access an element in the list by its alphabetize number, first write the proper noun of the list, and so in square brackets write the integer of the element's index.
For example, if yous wanted to access the element that has an index of 2, you lot'd do:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] print(names[2]) #output #Kenny
Lists in Python are mutable
In Python, when objects are mutable, it means that their values can be changed in one case they've been created.
Lists are mutable objects, so yous tin can update and change them after they accept been created.
Lists are also dynamic, significant they tin grow and compress throughout the life of a plan.
Items can be removed from an existing list, and new items can exist added to an existing list.
There are built-in methods for both adding and removing items from lists.
For example, to add items, in that location are the .append()
, .insert()
and .extend()
methods.
To remove items, there are the .remove()
, .pop()
and .pop(index)
methods.
What does the .suspend()
method do?
The .append()
method adds an additional element to the end of an already existing list.
The general syntax looks something like this:
list_name.append(item)
Let's break it downward:
-
list_name
is the proper noun you've given the list. -
.append()
is the list method for adding an item to the finish oflist_name
. -
item
is the specified individual item you want to add.
When using .append()
, the original list gets modified. No new list gets created.
If you wanted to add an extra name to the list created from earlier on, yous would do the following:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #add together the name Dylan to the cease of the list names.append("Dylan") print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny', 'Dylan']
What'due south the divergence between the .append()
and .insert()
methods?
The difference between the two methods is that .append()
adds an item to the end of a list, whereas .insert()
inserts and item in a specified position in the listing.
As you lot saw in the previous section, .suspend()
will add together the item you laissez passer as the argument to the part e'er to the end of the list.
If you don't want to just add items to the end of a list, you tin can specify the position you want to add them with .insert()
.
The general syntax looks similar this:
list_name.insert(position,item)
Permit'due south break it down:
-
list_name
is the name of the list. -
.insert()
is the list method for inserting an detail in a list. -
position
is the get-go statement to the method. It's e'er an integer - specifically it's the index number of the position where yous want the new item to be placed. -
item
is the second statement to the method. Here you specify the new item you want to add to the list.
For example, say yous had the following list of programming languages:
programming_languages = ["JavaScript", "Java", "C++"] print(programming_languages) #output #['JavaScript', 'Java', 'C++']
If you wanted to insert "Python" at the start of the listing, as a new item to the listing, you would utilize the .insert()
method and specify the position as 0
. (Remember that the first value in a listing always has an alphabetize of 0.)
programming_languages = ["JavaScript", "Java", "C++"] programming_languages.insert(0, "Python") print(programming_languages) #output #['Python', 'JavaScript', 'Java', 'C++']
If you instead had wanted "JavaScript" to be the starting time particular in the list, and so add together "Python" as the new detail, you would specify the position equally 1
:
programming_languages = ["JavaScript", "Java", "C++"] programming_languages.insert(1,"Python") impress(programming_languages) #output #['JavaScript', 'Python', 'Java', 'C++']
The .insert()
method gives yous a bit more flexibility compared to the .append()
method which only adds a new item to the end of the list.
What's the difference between the .append()
and .extend()
methods?
What if you want to add more than one item to a list at once, instead of adding them one at a time?
Y'all tin use the .suspend()
method to add more than one item to the terminate of a listing.
Say you have one list that contains only 2 programming languages:
programming_languages = ["JavaScript", "Coffee"] print(programming_languages) #output #['JavaScript', 'Coffee']
You and then want to add two more languages, at the stop of it.
In that example, you pass a listing containing the two new values you desire to add, as an argument to .append()
:
programming_languages = ["JavaScript", "Java"] #add two new items to the terminate of the list programming_languages.append(["Python","C++"]) print(programming_languages) #output #['JavaScript', 'Java', ['Python', 'C++']]
If you take a closer await at the output from higher up, ['JavaScript', 'Java', ['Python', 'C++']]
, y'all'll see that a new listing got added to the end of the already existing list.
And so, .append()
adds a list inside of a list.
Lists are objects, and when you use .append()
to add together another list into a listing, the new items will be added equally a single object (item).
Say y'all already had ii lists, like so:
names = ["Jimmy", "Timmy"] more_names = ["Kenny", "Lenny"]
What if wanted to combine the contents of both lists into one, by calculation the contents of more_names
to names
?
When the .append()
method is used for this purpose, another list gets created inside of names
:
names = ["Jimmy", "Timmy"] more_names = ["Kenny", "Lenny"] #add together contents of more_names to names names.append(more_names) impress(names) #output #['Jimmy', 'Timmy', ['Kenny', 'Lenny']]
So, .append()
adds the new elements as another listing, past appending the object to the terminate.
To actually concatenate (add) lists together, and combine all items from one list to another, you need to utilize the .extend()
method.
The full general syntax looks like this:
list_name.extend(iterable/other_list_name)
Let'south break it down:
-
list_name
is the proper noun of one of the lists. -
.extend()
is the method for adding all contents of one listing to another. -
iterable
can be any iterable such as some other list, for case,another_list_name
. In that case,another_list_name
is a list that will exist concatenated withlist_name
, and its contents volition be added i by one to the cease oflist_name
, equally split up items.
So, taking the case from earlier on, when .append()
is replaced with .extend()
, the output will wait like this:
names = ["Jimmy", "Timmy"] more_names = ["Kenny", "Lenny"] names.extend(more_names) print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny']
When nosotros used .extend()
, the names
list got extended and its length increased by 2.
The style .extend()
works is that it takes a listing (or other iterable) as an argument, iterates over each chemical element, and and then each element in the iterable gets added to the list.
There is some other difference between .suspend()
and .extend()
.
When you desire to add together a string, as seen earlier, .append()
adds the whole, single item to the finish of the list:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #add the name Dylan to the end of the list names.append("Dylan") print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny', 'Dylan']
If you lot used .extend()
instead to add together a string to the stop of a list ,each character in the cord would exist added as an individual particular to the list.
This is considering strings are an iterable, and .extend()
iterates over the iterable statement passed to information technology.
And so, the example from to a higher place would look similar this:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #pass a string(iterable) to .extend() names.extend("Dylan") impress(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny', 'D', 'y', 'l', 'a', 'due north']
Conclusion
To sum up, the .append()
method is used for adding an item to the terminate of an existing list, without creating a new list.
When it is used for adding a list to some other list, it creates a list within a listing.
If y'all want to larn more about Python, check out freeCodeCamp's Python Certification. You'll kickoff learning in an interacitve and beginner-friendly manner. You'll also build five projects at the end to put into practice what you learned.
Cheers for reading and happy coding!
Learn to code for complimentary. freeCodeCamp's open source curriculum has helped more than twoscore,000 people get jobs as developers. Become started
How To Add A Single Item To An Array In Java,
Source: https://www.freecodecamp.org/news/append-in-python-how-to-append-to-a-list-or-an-array/
Posted by: scottuporthe.blogspot.com
0 Response to "How To Add A Single Item To An Array In Java"
Post a Comment